How to use the new response API from OpenAI API
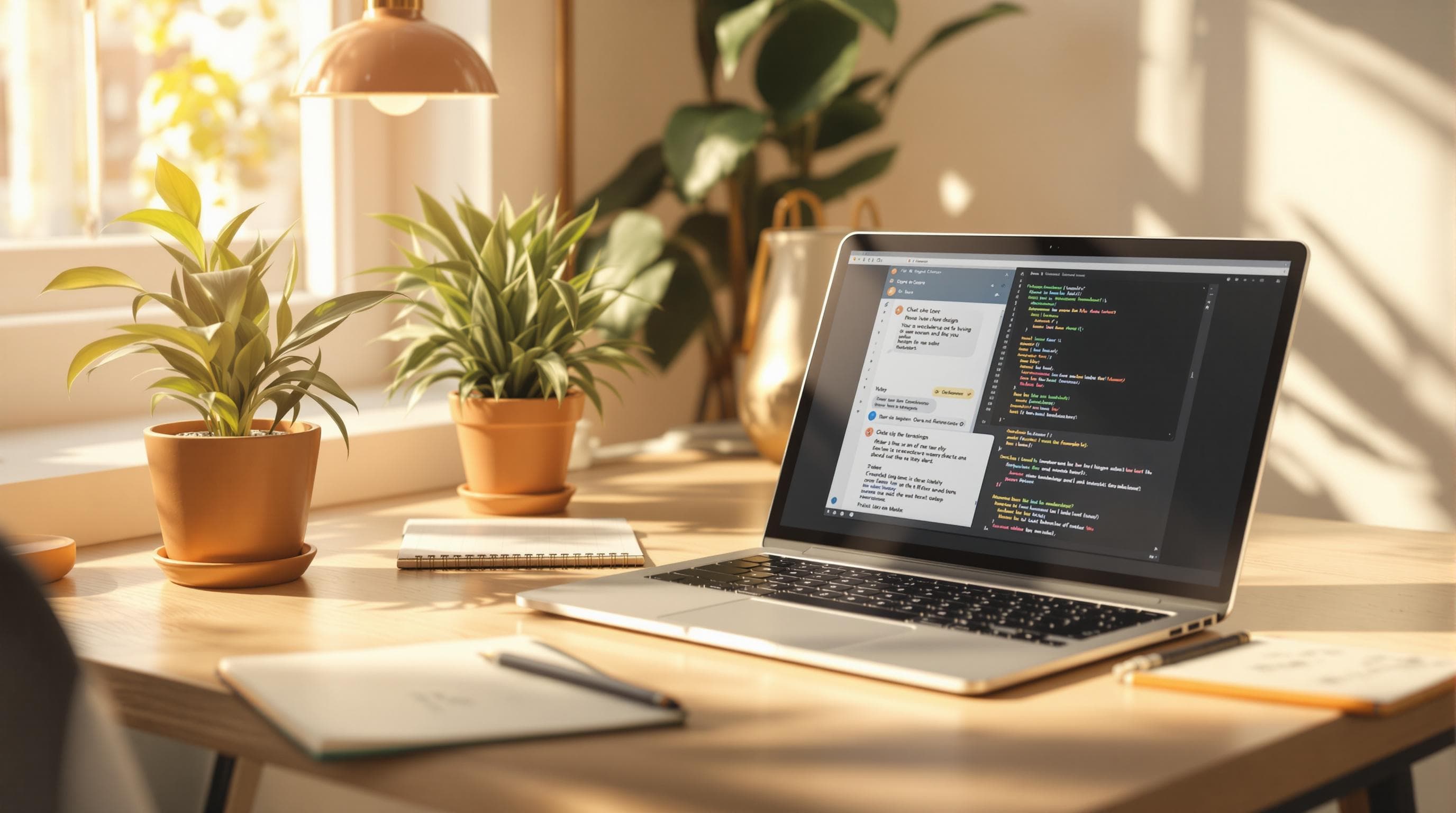
How to use the new response API from OpenAI API
The OpenAI Response API is designed to simplify ai agent development while offering advanced features for better performance and control. Here's what you can expect:
- Automatic context management: Handles multi-turn conversations without manual state tracking.
- Structured and consistent replies: Outputs are easy to parse, with support for code blocks and structured data.
- Faster token processing: Optimized for high-traffic use cases.
- Tool integrations: Seamlessly connect external tools like weather APIs or document analyzers.
- Enhanced error handling: Clearer error messages and robust retry mechanisms.
Quick Start:
-
Setup: Install the OpenAI Node.js client and configure your API key in a
.env
file. - First Request: Test basic chatbot functionality with minimal setup.
- Advanced Features: Use streaming, external tool integrations, or document analysis for complex tasks.
Feature | Chat Completions API | Response API |
---|---|---|
Context Management | Manual | Automatic |
Output Format | Basic | Structured |
Streaming Support | Limited | Enhanced |
Error Handling | Basic | Detailed |
Tool Integrations | No | Yes |
Switching from older APIs is straightforward, and the Response API ensures compatibility with existing systems. Whether you're building chatbots from scratch or enhancing existing ones, this API offers the tools you need for efficient, scalable conversational AI.
OpenAI Responses API Tutorial #1 - Getting Started
Setup Requirements
Before you can use the OpenAI Response API, you’ll need to prepare your development environment.
Accounts and Keys You’ll Need
Here’s what you’ll need to get started:
- OpenAI Account: Sign up at platform.openai.com.
- API Key: Generate an API key from the OpenAI dashboard.
- Organization ID: Locate your organization ID in the account settings.
To keep your API key secure, store it as an environment variable instead of embedding it in your code. Create a .env
file in your project’s root directory and add the following:
OPENAI_API_KEY=your-api-key-here
OPENAI_ORG_ID=your-org-id-here
Make sure to rotate keys periodically and monitor their usage via the OpenAI dashboard.
Installation Steps
Start by setting up a new project directory:
mkdir response-api-project
cd response-api-project
npm init -y
Then, install the OpenAI Node.js client and dotenv for managing environment variables:
npm install openai@^1.12.0 dotenv
Configuring Your Development Environment
Organize your project structure like this:
response-api-project/
├── .env
├── package.json
├── src/
│ └── index.js
└── .gitignore
Now, initialize the OpenAI client. Add the following code to src/index.js
:
require('dotenv').config();
const OpenAI = require('openai');
const openai = new OpenAI({
apiKey: process.env.OPENAI_API_KEY,
organization: process.env.OPENAI_ORG_ID
});
Verifying Your Setup
To ensure everything is working, test the setup with a basic API call. Add this function to your src/index.js
file:
async function testSetup() {
try {
const response = await openai.completions.create({
model: "gpt-4",
messages: [{ role: "user", content: "Hello!" }]
});
console.log('Setup successful!');
} catch (error) {
console.error('Setup error:', error.message);
}
}
Run the script to confirm your setup is complete. If everything is configured properly, you’ll see a success message in your console.
Main API Features
The Response API simplifies chatbot development with a range of powerful features. Let’s dive into its main capabilities:
External Tool Integration
The Response API allows integration with external tools using function calls. Here's an example of how to set it up:
const response = await openai.beta.responses.create({
model: "gpt-4",
messages: [{
role: "user",
content: "What's the weather in New York?"
}],
tools: [{
type: "function",
function: {
name: "get_weather",
description: "Get current weather data for a location",
parameters: {
type: "object",
properties: {
location: {
type: "string",
description: "City name"
}
},
required: ["location"]
}
}
}]
});
This setup enables seamless coordination between services, making workflows smoother. Now, let’s look at how the API handles document search and analysis.
Search and Document Analysis
The Response API supports document analysis and search, making it easier to process and understand file content. Here’s an example:
const response = await openai.beta.responses.create({
model: "gpt-4",
messages: [{
role: "user",
content: "Analyze this PDF document"
}],
file_ids: ["file-xyz123"],
max_tokens: 1000
});
Supported file formats include:
Format | Max File Size | Supported Features |
---|---|---|
512 MB | Text, Tables, Images | |
TXT | 100 MB | Plain Text |
DOCX | 256 MB | Formatted Text, Tables |
JSON | 50 MB | Structured Data |
These features make it easy to work with different file types while extracting useful insights. Let’s move on to how the API manages chat history.
Chat History Management
Maintaining conversation context is crucial for effective chatbot interactions. The API offers tools for advanced chat history management:
const conversation = await openai.beta.responses.create({
model: "gpt-4",
messages: [
{
role: "system",
content: "Maintain context for technical support"
},
{
role: "user",
content: "Previous message content"
},
{
role: "assistant",
content: "Earlier response"
}
],
context_window: 4096,
memory_type: "conversation"
});
With features like token limit management, context retention, and support for multi-user scenarios, the API ensures smooth, context-aware interactions.
sbb-itb-7a6b5a0
Using the Response API
First API Request
Start with a simple request using the Response API:
const openai = require('openai');
const client = new openai({ apiKey: process.env.OPENAI_API_KEY });
async function makeBasicRequest() {
try {
const response = await client.beta.responses.create({
model: "gpt-4",
messages: [{
role: "user",
content: "Generate a summary of climate change impacts"
}],
temperature: 0.7,
max_tokens: 500
});
console.log(response.choices[0].message.content);
} catch (error) {
console.error('Error:', error.message);
}
}
Once you've tested this, you can move on to more advanced configurations for greater control.
Advanced Configuration
The Response API includes options to fine-tune your requests:
const response = await client.beta.responses.create({
model: "gpt-4",
messages: [{
role: "user",
content: "Analyze market trends"
}],
stream: true,
temperature: 0.4,
presence_penalty: 0.6,
frequency_penalty: 0.3,
response_format: { type: "json_object" }
});
for await (const chunk of response) {
process.stdout.write(chunk.choices[0]?.delta?.content || '');
}
Parameter | Value Range | Description |
---|---|---|
temperature | 0.0 - 1.0 | Adjusts randomness in responses |
max_tokens | 1 - 4096 | Limits the response length |
presence_penalty | -2.0 - 2.0 | Discourages repeating topics |
frequency_penalty | -2.0 - 2.0 | Reduces repeated tokens |
response_format | json_object, text | Specifies the output format type |
Testing and Error Handling
After setting up advanced configurations, ensure your implementation can handle errors effectively:
async function handleAPIRequest() {
try {
const response = await client.beta.responses.create({
model: "gpt-4",
messages: [{
role: "user",
content: "Process this request"
}]
});
return response;
} catch (error) {
if (error.status === 429) {
// Rate limit exceeded
await delay(1000);
return handleAPIRequest();
} else if (error.status === 401) {
// Authentication error
throw new Error('Invalid API key');
} else if (error.status === 400) {
// Invalid request
console.error('Request validation failed:', error.message);
} else {
// Generic error handling
console.error('Unexpected error:', error.message);
}
}
}
Here’s a quick guide to common error codes and how to resolve them:
Error Code | Description | Resolution |
---|---|---|
429 | Rate Limit | Use exponential backoff |
401 | Authentication | Verify your API key |
400 | Invalid Request | Check your request parameters |
500 | Server Error | Retry using a backoff strategy |
503 | Service Unavailable | Consider implementing a circuit breaker |
To avoid common validation issues, you can use a helper function like this:
function validateRequest(messages) {
if (!Array.isArray(messages) || messages.length === 0) {
throw new Error('Messages array cannot be empty');
}
messages.forEach(msg => {
if (!msg.role || !msg.content) {
throw new Error('Invalid message format');
}
});
return true;
}
Switching from Chat Completions
API Changes Overview
Migrating from the Chat Completions API to the Response API is simple and comes with new features while keeping the core functionality familiar.
// Old Chat Completions API
const completion = await openai.chat.completions.create({
model: "gpt-4",
messages: messages
});
// New Response API
const response = await openai.beta.responses.create({
model: "gpt-4",
messages: messages
});
Here’s a quick comparison of the two APIs:
Feature | Chat Completions | Response API |
---|---|---|
Base Endpoint | /v1/chat/completions |
/v1/beta/responses |
Response Format | Single message format | Enhanced structured output |
Streaming Support | Basic streaming | Better chunk handling |
Error Handling | Basic error codes | Detailed error messages |
Token Usage | Simple count | Detailed usage breakdown |
Below are the steps to update your implementation.
Update Instructions
1. Dependencies
Ensure your openai
library is updated to the required version:
{
"dependencies": {
"openai": "^1.12.0" // Minimum version required
}
}
2. API Implementation
Reinitialize your client and adjust your API calls:
// Reinitialize your client
const client = new openai({
apiKey: process.env.OPENAI_API_KEY,
defaultHeaders: {
'OpenAI-Beta': 'responses=v1'
}
});
async function generateResponse(prompt) {
const response = await client.beta.responses.create({
model: "gpt-4",
messages: [{
role: "user",
content: prompt
}],
response_format: {
type: "text" // or "json_object" for structured output
}
});
return response.choices[0].message.content;
}
3. Stream Handling
For streaming, the new API offers improved chunk processing:
const stream = await client.beta.responses.create({
model: "gpt-4",
messages: messages,
stream: true
});
for await (const chunk of stream) {
const content = chunk.choices[0]?.delta?.content || '';
// Process streaming content
}
4. Error Handling
The Response API provides more detailed error codes and messages:
try {
const response = await client.beta.responses.create({
model: "gpt-4",
messages: messages
});
} catch (error) {
if (error.code === 'responses_not_enabled') {
console.error('Response API access not enabled for your account');
} else if (error.code === 'invalid_response_format') {
console.error('Response format configuration error');
}
}
Usage Guidelines
Cost and Performance Tips
Make the most out of the API while keeping expenses low:
const response = await client.beta.responses.create({
model: "gpt-4",
messages: messages,
max_tokens: 150,
temperature: 0.7,
presence_penalty: 0.6,
frequency_penalty: 0.1
});
Here are some ways to manage costs and improve efficiency:
- Token Management: Use a token counter to monitor usage. Keep prompts short and focused.
- Caching Strategy: Store responses for frequently asked questions to avoid repeating the same requests.
- Batch Requests: Group similar requests together to cut down on overhead.
-
Response Format: Only use
json_object
format when structured data is absolutely necessary.
Don't overlook the importance of securing your API setup.
API Security
Keep your API implementation secure with these practices:
const keyManager = {
primary: process.env.OPENAI_API_KEY,
secondary: process.env.OPENAI_API_KEY_BACKUP,
rotationInterval: 7 * 24 * 60 * 60 * 1000 // 7 days
};
const rateLimit = {
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
};
Key steps to enhance security:
- Use request rate limiting to prevent abuse.
- Always communicate via HTTPS to protect data in transit.
- Rotate API keys regularly to minimize risks.
- Watch for unusual usage patterns that could signal misuse.
Model Selection
Once performance and security are addressed, choose the model that fits your needs:
Model | Best For | Response Time | Token Limit |
---|---|---|---|
GPT-4 | Complex reasoning, code generation | 3-5 seconds | 8,192 tokens |
GPT-3.5-turbo | General-purpose, chat | 0.5-1 second | 4,096 tokens |
GPT-3.5-turbo-16k | Long-form content | 1-2 seconds | 16,384 tokens |
Consider the following when picking a model:
- Task complexity and reasoning needs
- How quickly you need responses
- Input/output length requirements
- Budget limitations
- Desired accuracy
Choosing the right model for your specific use case helps balance performance and cost. Using a higher-powered model than necessary can drive up expenses without adding much value.
Conclusion
The Response API takes AI integration to the next level, providing better control and efficiency. With features like effective token management, strong security measures, and smart model selection, it helps create reliable and budget-friendly AI applications that consistently perform well.
It also supports simpler solutions like OpenAssistantGPT, a no-code platform using the OpenAI Assistant API. OpenAssistantGPT allows quick chatbot setup with advanced tools such as web crawling and file analysis. Pricing starts at $18 per month and includes enterprise-level SAML/SSO security.
With improved context handling, advanced error management, and smooth integration options, the Response API serves as a strong base for custom projects or platform-based solutions. Whether you choose the direct API route or a user-friendly platform, you can achieve secure, scalable, and cost-efficient AI integration.